Blog
The main difference Codeigniter3 Vs Codeigniter4
- January 28, 2023
- Posted by: techjediadmin
- Category: Web application Web Development
CodeIgniter 3 and CodeIgniter 4 are both PHP web application frameworks, but there are some key differences between the two versions:
Architecture: CodeIgniter 4 has a new modern architecture, which is more modular and flexible than CodeIgniter 3. It uses namespaces and autoloading, making it easier to organize and manage code.
Dependencies: CodeIgniter 4 requires PHP 7.2 or higher, while CodeIgniter 3 is compatible with PHP 5.6 and higher. CodeIgniter 4 also uses Composer to manage dependencies, while CodeIgniter 3 does not.
Router: CodeIgniter 4 has a new router that is more powerful and flexible than the router in CodeIgniter 3. It supports RESTful routes, and it’s easier to define and manage routes.
Database: CodeIgniter 4 has a new database abstraction layer that is more powerful and flexible than the one in CodeIgniter 3. It supports multiple database drivers and has more advanced query builder features.
Namespaces: CodeIgniter 4 uses namespaces and PSR-4 autoloading, which makes it easier to organize and manage code.
Model-View-Controller (MVC) Support: CodeIgniter 4 has improved support for the MVC pattern, making it easier to organize code and separate concerns.
Community: CodeIgniter 4 is relatively new and has a smaller community than CodeIgniter 3, which means that there are fewer resources and third-party packages available for it.
In summary, CodeIgniter 4 is a more modern and powerful version of CodeIgniter 3, but it requires a more recent version of PHP, and it has a smaller community. If you are starting a new project and want to take advantage of the latest features and best practices, CodeIgniter 4 is a good choice, but if you have an existing CodeIgniter 3 project, it might be easier to continue using it.
How to Install CodeIgniter 4
Ref: https://codeigniter.com/user_guide/intro/index.html
Here are some examples of how to use CodeIgniter 4:
Creating a controller: In CodeIgniter 4, controllers are classes that handle the logic for specific pages or sections of the website. Here is an example of how to create a controller:
<?php
namespace App\Controllers;
use CodeIgniter\Controller;
class News extends Controller
{
public function index()
{
// code to handle displaying a list of news articles
}
public function view($id)
{
// code to handle displaying a specific news article
}
}
Creating a model: In CodeIgniter 4, models are classes that handle the logic for interacting with the database. Here is an example of how to create a model:
<?php
namespace App\Models;
use CodeIgniter\Model;
class NewsModel extends Model
{
protected $table = 'news';
public function getNews($id)
{
return $this->find($id);
}
public function getAllNews()
{
return $this->findAll();
}
}
Creating a view: In CodeIgniter 4, views are templates that handle the presentation of the data. Here is an example of how to create a view:
<h1>News</h1>
<ul>
<?php foreach($news as $article): ?>
<li>
<h2><?= $article['title'] ?></h2>
<p><?= $article['content'] ?></p>
</li>
<?php endforeach; ?>
</ul>
Creating a Route: In CodeIgniter 4, routes are used to map URLs to specific controllers and methods. Here is an example of how to create a route:
$routes->get('news', 'News::index');
$routes->get('news/(:segment)', 'News::view/$1');
This route maps the URL /news to the index method of the News controller, and the URL /news/{id} to the view method of the News controller, where {id} is passed as a parameter.
It can be some of the best time you spend with this article. Happy reading!
Read Similar Blogs:
Databases — Running multi-line scripts from command line
Google Authenticator
How does Serverless Architecture work?
Courses
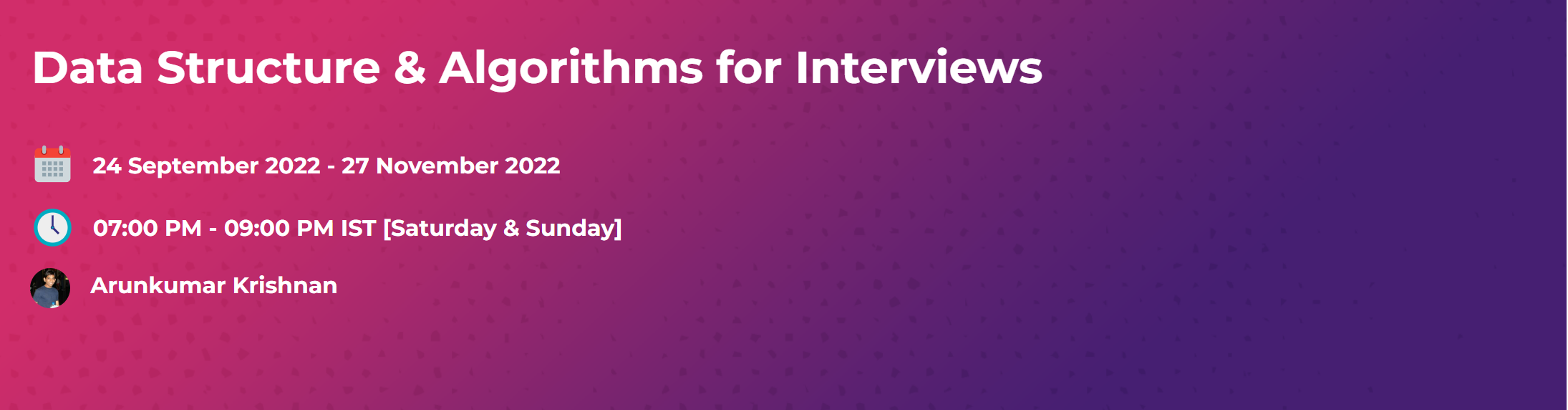
Data Structure & Algorithms for Interviews
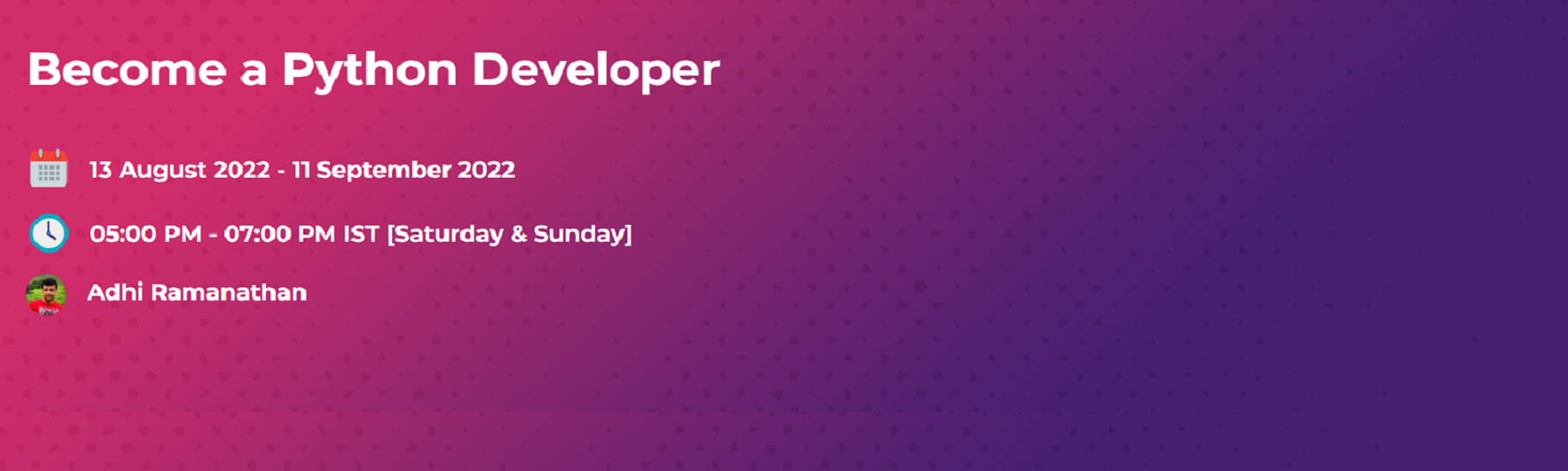